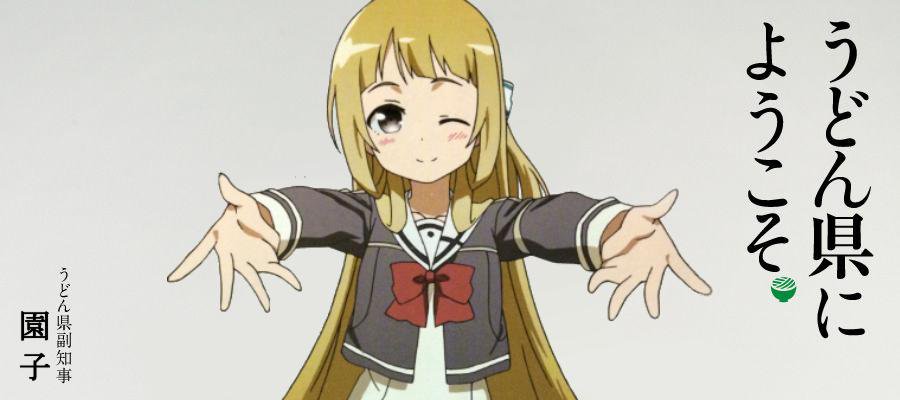
Android Library
To build a android library project, simply change the following line in build.gradle (Module):
apply plugin: 'com.android.application'
to:
apply plugin: 'com.android.library'
To pack the library into a jar file, add these lines to build.gradle (Module):
def JarLibName="YourLibName"
task clearJar(type: Delete) {
delete 'build/generated/' + JarLibName + '.jar'
}
task makeJar(type: Jar) {
from zipTree(file('build/intermediates/bundles/release/classes.jar'))
baseName = JarLibName
destinationDir = file("build/generated")
}
makeJar.dependsOn(clearJar, build)
And run the following command in the Terminal:
./gradlew makeJar
If failed in lint process, add these to build.gradle (Module):
android {
// ...
lintOptions {
abortOnError false
}
// ...
}
Remember to copy the jar library file to the "libs/" folder in your project.
For more infomation, refer to Packing Jar in Android Studio.
Proguard
To enable proguard and minify, add the following lines to build.gradle (Module):
android {
// ...
buildTypes {
release {
debuggable false
minifyEnabled true
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
}
}
// ...
}
Then, modify "proguard-rules.pro" file under your module path. Keep the class you wanna expose to outside.
For instance:
-keep class yourclassname {
public *;
}
-keep interface yourclassname$interfacename {
*;
}
-keep class yourclassname$innerclassname {
public static final java.lang.String SOME_CONSTANT;
public int some_function(java.lang.String);
}
For more detail, refer to ProGuard in Android.